NeighborhoodMatch
NeighborhoodMatch returns a set of nearby neighborhoods most similar to the current neighborhood in terms of qualities like Local Logic lifestyle scores, demographics, and housing composition.
At setup, customers provide an eligible list of neighborhoods to match against. This list should include a link to an image and a link to a landing page for each neighborhood.
Only neighborhoods from that list within the same metro area as the current neighborhood are eligible for matching. If the current neighborhood is not within a metro area, then we will match against eligible neighborhoods within 100 kilometers of the target neighborhood.
For more details on the similarity score and how neighborhoods are calculated, see the "Calculating Neighborhood Similarity" of the Similar Neighborhoods API endpoint documentation.
The affordability index (price comparison tag) is only available in the US.
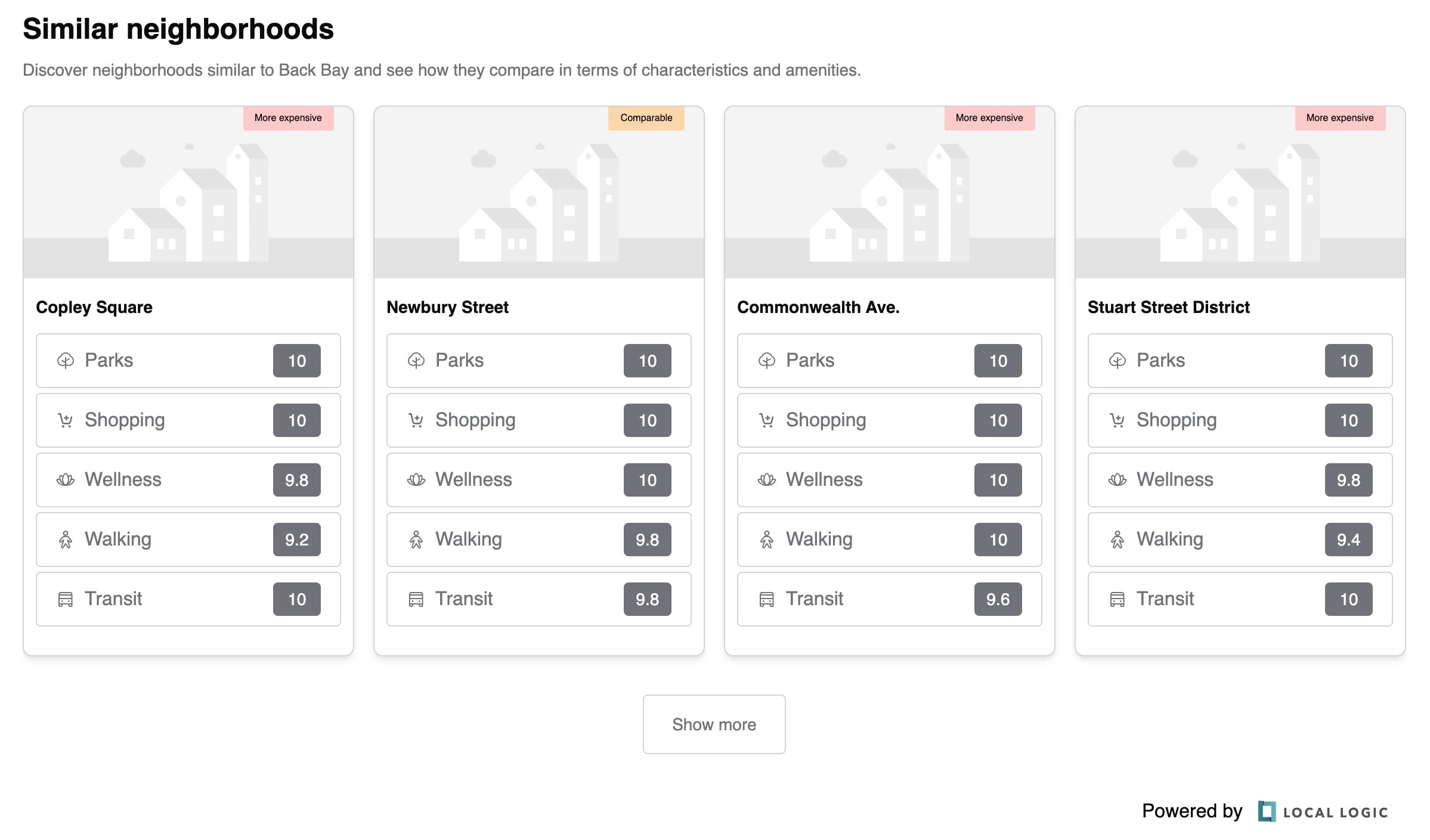
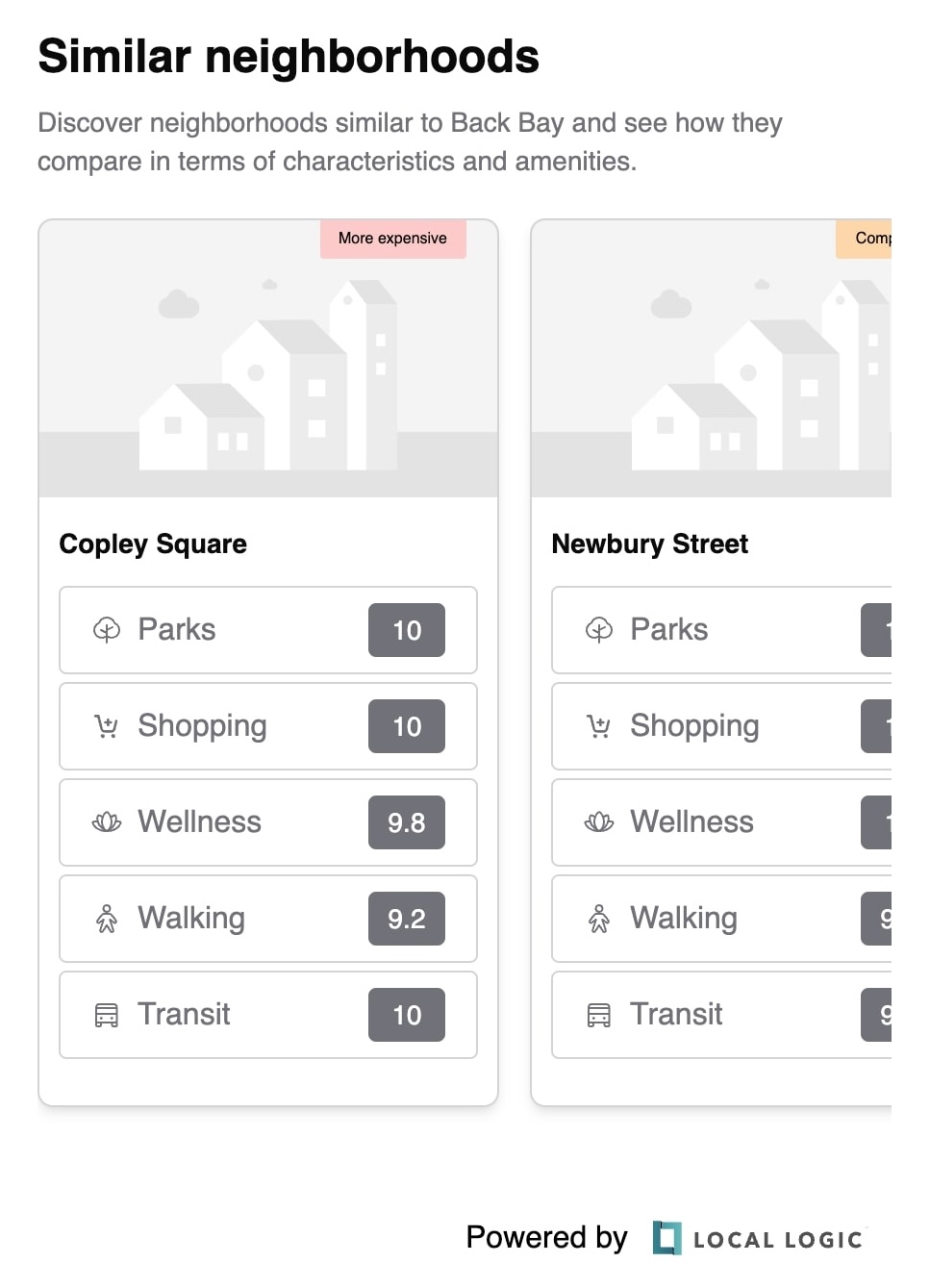
Installation
Our SDK products are served from a single source.
- React
- React Native
- Vanilla JavaScript
The SDKs are designed to work with React and other declarative frameworks.
First, install the entry script with npm
, yarn
, or pnpm
:
npm i --save @local-logic/sdks-js
yarn add @local-logic/sdks-js
pnpm add @local-logic/sdks-js
Example
import { useRef, useEffect } from "react";
import LLSDKsJS from "@local-logic/sdks-js";
const globalOptions = {
locale: "en", // If available, change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
const localLogicClient = LLSDKsJS("your-api-key-here", globalOptions);
function NeighborhoodMatchSDKC#omponent() {
const containerRef = useRef(null);
useEffect(() => {
if (!containerRef.current) {
return;
}
const sdkOptions = {
geographyId: "g10_drt2yt86"
// ...Other sdk specific options
}
const sdkInstance = localLogicClient.create(
"neighborhood-match",
containerRef.current,
sdkOptions,
);
// It is important to destroy the SDK instance when the component is unmounted.
return () => {
sdkInstance.destroy();
}
}, []);
return (
<div
ref={containerRef}
style={{
// Height is dependent on the SDK you are implementing
height: "100%",
width: "100%",
}}
/>
);
}
export default NeighborhoodMatchSDKC#omponent;
The Local Logic React Native SDK component allows you to easily add one of our SDKs to your native Android and iOS apps using React Native.
First, install the entry script with npm
, yarn
, or pnpm
:
npm i --save @local-logic/sdks-js
yarn add @local-logic/sdks-js
pnpm add @local-logic/sdks-js
Install React Native Webview dependency
React Native Webview is used to port Local Logic SDKs to React Native.
Expo CLI
Install supported react-native-webview
version for Expo applications using Expo CLI.
npx expo install react-native-webview
React Native CLI
Install react-native-webview
using your project's package manager:
npm i --save react-native-webview
yarn add react-native-webview
pnpm add react-native-webview
If using CocoaPods, in the ios/
or macos/
directory run:
pod install
Usage
The React Native component accepts the same options as our web SDKs via it's options
, appearanceOptions
, and renderOptions
props.
import LLSDKs from "@local-logic/sdks-react-native";
const globalOptions = {
locale: "en", // Change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
const sdkOptions = {
geographyId: "g10_drt2yt86"
// ...Other sdk specific options
};
<LLSDKs
apiKey="your-api-key-here"
sdkType="neighborhood-match"
options={sdkOptions}
appearanceOptions={globalOptions}
renderOptions={{ lazy: false }}
/>
The script is available in umd
and es
format from the Local Logic CDN: https://sdk.locallogic.co/sdks-js/<VERSION>/index.<FORMAT>.js
<VERSION>
is the latest script version number (which you can find on npm), and <FORMAT>
is either umd
or es
.
Example
<!DOCTYPE html>
<html>
<head>
<title>SDK Javascript Example</title>
<meta charset="UTF-8" />
</head>
<body>
<script
async
src="https://sdk.locallogic.co/sdks-js/<version>/index.umd.js"
onload="loadNeighborhoodMatchSDK()"
></script>
<style>
#neighborhood-match-widget {
height: 100%;
width: 100%;
}
</style>
<!--NOTE: If you are implementing multiple SDKs, make sure you create unique IDs for each-->
<div id="neighborhood-match-widget"></div>
<script>
const globalOptions = {
locale: "en", // Change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
function loadNeighborhoodMatchSDK() {
// Your API key or token
const ll = LLSDKsJS("your-api-key-here", globalOptions);
// This is the div that will contain the widget
const sdkContainer = document.getElementById("neighborhood-match-widget");
const sdkOptions = {
geographyId: "g10_drt2yt86"
// ...Other sdk specific options
}
const sdkInstance = ll.create("neighborhood-match", sdkContainer, sdkOptions);
}
</script>
</body>
</html>
Configuration
API Key
Your API Key will be provided to you by the LocalLogic team.
Neighborhoods
As part of the NeighborhoodMatch SDK, you have the option to customize the implementation in three ways:
-
Restrict the returned similar neighborhoods to only neighborhoods relevant to you.
This is important because it allows you to keep the homeseeker in your funnel by not recommending neighborhoods outside of your territory or where you do not have a page. By default, when no configuration is provided “all” similar nearby neighborhoods are returned; the only filtering applied is to limit to other similar neighborhoods within the metropolitan statistical area (MSA) or within 100 kms, if the neighborhood is not within an MSA.
-
Customize the images displayed for each neighborhood.
This allows you to avoid prescribed images. By default, no images will be shown for neighborhoods without configured images.
-
Assign each similar neighborhood card to a link.
The vision here is that similar neighborhoods can be used to “shop” between neighborhoods by directly linking neighborhood pages (either NeighborhoodWrap implementations or even as an addition to non-NeighborhoodWrap neighborhood pages). The goal of recommending similar neighborhoods is to allow a user to click through and learn about the neighborhood and view homes in the area. For this to work, links must be assigned for when a user clicks on each card. By default, no link is added to cards without a link configured: they can be viewed but not clicked.
For the NeighborhoodMatch SDK, a configuration file is leveraged to customize the list of neighborhoods used and displayed to the user, to ensure they link within the implementing site’s available pages, and to customize the images displayed for each neighborhood.
When no configuration file is provided, no images will be shown for each neighborhood, the list of similar neighborhoods will not be filtered, and the cards in the SDK will not display as links. However, the SDK can still be implemented without the configuration being applied and it will be updated to match the given neighborhood once the configuration file is applied.
Generating a NeighborhoodMatch Configuration File
- Prepare a CSV file that contains the fields outlined below.
- Send the CSV to Local Logic via a support ticket. The team at Local Logic will help convert the neighborhoods to supported geog_id’s and apply the configuration the next business day.
CSV Information
Example configuration file - geog_id
Example configuration file - neighborhood name
Field | Required | Description |
---|---|---|
geog_id OR neighborhood | true | geog_id is the specific Local Logic external ID for the neighborhood, if available and known. neighborhood is the name of the neighborhood for identifying and matching to our Local Logic list of neighborhoods. |
thumbnail_url | true | The full URL for the image representing the neighborhood when it appears in configured NeighborhoodMatch SDKs. |
redirect_url | true | The full URL that should be the source link if a user clicks on the neighborhood. It should be a URL on your site that exists (or will exist) for each neighborhood. |
The "neighborhood" option can be used if the Local Logic geog_id is unknown for the desired neighborhood. In this case, be as descriptive as possible: “Little Italy, San Diego, CA” is better than “Little Italy,” as there are at least 8 Little Italies in the United States.
For the "thumbnail_url", the server where the image thumbnail URL is hosted needs at a minimum to have the following addresses added as allowed origins to the CORS header:
-
Required for the SDK implementation:
https://sdk.locallogic.co/
-
Highly recommended for support, testing, and debugging:
https://staging.locallogic.co/
http://localhost:3000
http://localhost:3001
The thumbnail image could also be publicly accessible (if CORS headers are not used).
It’s recommended that your thumbnail image(s) be at minimum 144 px by 400 px and at maximum 500 px by 800 px for best results in the SDK.
Global options
const localLogicClient = localLogicSDK(apiKey, globalOptions)
These options are available accross SDKs. You will also be able to specify SDK specific options depending on the SDK you are implementing. These options will be available in the SDK specific documentation
Name | Required | Type | Default | Description |
---|---|---|---|---|
apiKey | true | string | ApiKey required for making requests to the Local Logic API. | |
globalOptions.appearance | false | Appearance | The appearance option provides theme and variable support customizing the look and feel of your widgets. | |
globalOptions.locale | false | "en" or "fr" | "en" | The locale option specifies the language of the scores and the UI interface. |
globalOptions.externalId | false | string | For SDKs connected to the Neighborhood Management App in the Local Logic Hub. This field is required for Neighborhood Showcase and Favorite Neighborhoods SDKs to work |
Appearance
Local Logic SDKs support visual customization using the Appearance API, which allows you to match the look of the SDK to your brand.
type Appearance = {
theme?: "day" | "night"; // Defaults to "day"
variables?: {
[key: string]: string;
};
}
Commonly used variables
Variable | Description |
---|---|
--ll-color-primary | The primary brand color. |
--ll-color-primary-variant1 | A slightly darker version of the primary brand colour. This variable should always be changed in conjunction with --ll-color-primary . |
--ll-font-family | Changes the font family used throughout the SDKs. Currently, the SDKs only support system fonts. This value should always include a fallback font family, ex. Inter, sans-serif . |
--ll-font-size-base | Used to scale the font size up or down. |
--ll-spacing-base-unit | Used to scale the overall padding of the SDKs. |
Functions
Once you initialize your SDK client with your API key and global options, several functions are available.
Create
const sdkInstance = localLogicClient.create("neighborhood-match", container, sdkOptions)
This function creates a new SDK widget based on the name specified.
sdkOptions
are specific to each SDK being created.
Name | Required | Type | Default | Description |
---|---|---|---|---|
sdkType | true | string | The SDK you would like to create. "neighborhood-match" in this case. | |
container | true | HTMLElement | The element to render in to. | |
sdkOptions | true | SDKOptions | Options required for te specified sdkType . Options are detailed below. |
SDKOptions
Name | Required | Type | Default | Description |
---|---|---|---|---|
sdkOptions.geographyId | * | string | The Local Logic geography ID. This is the preferred instantiation method. * This field is required if you are not using lat/lng . | |
sdkOptions.lat | * | number | Initial viewport latitude. * This field is required if you are not using geographyId . If geographyId is defined, this field is ignored. | |
sdkOptions.lng | * | number | Initial viewport longitude. * This field is required if you are not using geographyId . If geographyId is defined, this field is ignored. | |
sdkOptions.title | false | boolean | string | Title is enabled by default and is provided as a translated string. You have the ability to remove the title or supply your own title string. | |
sdkOptions.hideAffordabilityTags | false | boolean | false | Specifies whether or not the affordability comparison tag on each neighborhood card should be hidden. |
sdkOptions.hideThumbnails | false | boolean | false | Specifies whether or not the neighborhood image on each neighborhood card should be hidden. No image will be displayed if this is set to true. |
Update
sdkInstance.update(sdkOptions)
This function is used to update the widget with new values. This can be useful when, for example, you want to change the widget location.
The sdkOptions
object follows the same structure as on creation.
On
sdkInstance.on(event, callback)
This function takes a callback which is triggered when an event
occurs. Currently, there is only one event.
Name | Required | Type | Default | Description |
---|---|---|---|---|
event | true | "change" | The name of the event . | |
callback | true | CallbackFunction | The callback to be triggered when the specified event occurs. |
type CallbackFunction = ({ type: string, data: unknown }) => void;
Destroy
sdkInstance.destroy()
This function is used to teardown the created SDK widget.
Supporting API Endpoints
The following Local Logic APIs are used by the SDK to display data.
API | Description |
---|---|
Similar Neighborhoods | Used to retrieve the configured client-specific neighborhoods with the highest similarity to the given neighborhood. |