NeighborhoodSchools
NeighborhoodSchools display a table of schools based on their proximity to the location.
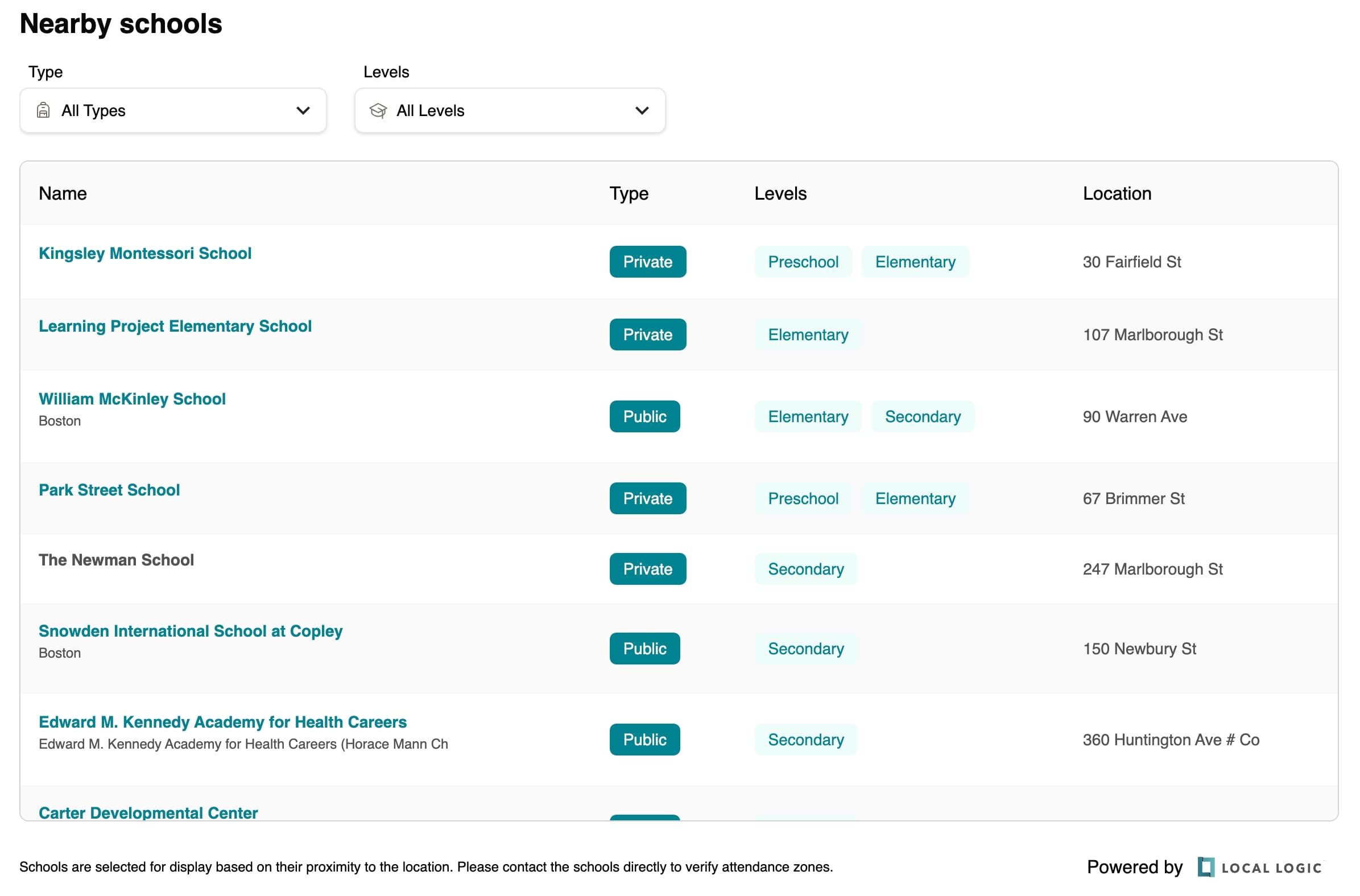
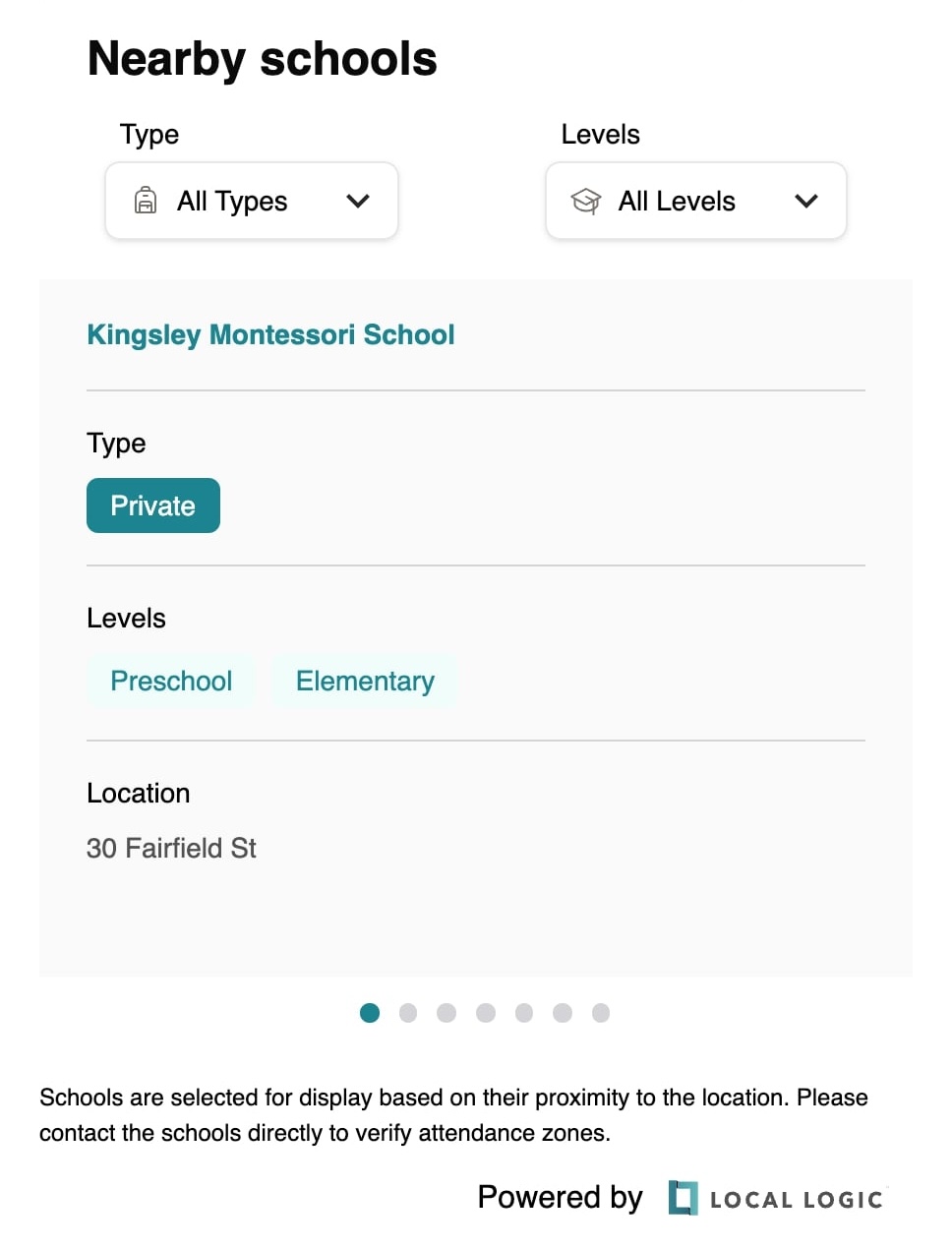
Installationβ
Our SDK products are served from a single source.
- React
- React Native
- Vanilla JavaScript
The SDKs are designed to work with React and other declarative frameworks.
First, install the entry script with npm
, yarn
, or pnpm
:
npm i --save @local-logic/sdks-js
yarn add @local-logic/sdks-js
pnpm add @local-logic/sdks-js
Example
import { useRef, useEffect } from "react";
import LLSDKsJS from "@local-logic/sdks-js";
const globalOptions = {
locale: "en", // If available, change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
const localLogicClient = LLSDKsJS("your-api-key-here", globalOptions);
function NeighborhoodSchoolsSDKComponent() {
const containerRef = useRef(null);
useEffect(() => {
if (!containerRef.current) {
return;
}
const sdkOptions = {
geographyId: "g10_drt2yt86"
// ...Other sdk specific options
}
const sdkInstance = localLogicClient.create(
"neighborhood-schools",
containerRef.current,
sdkOptions,
);
// It is important to destroy the SDK instance when the component is unmounted.
return () => {
sdkInstance.destroy();
}
}, []);
return (
<div
ref={containerRef}
style={{
// Height is dependent on the SDK you are implementing
height: "700px",
width: "100%",
}}
/>
);
}
export default NeighborhoodSchoolsSDKComponent;
The Local Logic React Native SDK component allows you to easily add one of our SDKs to your native Android and iOS apps using React Native.
First, install the entry script with npm
, yarn
, or pnpm
:
npm i --save @local-logic/sdks-js
yarn add @local-logic/sdks-js
pnpm add @local-logic/sdks-js
Install React Native Webview dependency
React Native Webview is used to port Local Logic SDKs to React Native.
Expo CLI
Install supported react-native-webview
version for Expo applications using Expo CLI.
npx expo install react-native-webview
React Native CLI
Install react-native-webview
using your project's package manager:
npm i --save react-native-webview
yarn add react-native-webview
pnpm add react-native-webview
If using CocoaPods, in the ios/
or macos/
directory run:
pod install
Usage
The React Native component accepts the same options as our web SDKs via it's options
, appearanceOptions
, and renderOptions
props.
import LLSDKs from "@local-logic/sdks-react-native";
const globalOptions = {
locale: "en", // Change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
const sdkOptions = {
geographyId: "g10_drt2yt86"
// ...Other sdk specific options
};
<LLSDKs
apiKey="your-api-key-here"
sdkType="neighborhood-schools"
options={sdkOptions}
appearanceOptions={globalOptions}
renderOptions={{ lazy: false }}
/>
The script is available in umd
and es
format from the Local Logic CDN: https://sdk.locallogic.co/sdks-js/<VERSION>/index.<FORMAT>.js
<VERSION>
is the latest script version number (which you can find on npm), and <FORMAT>
is either umd
or es
.
Example
<!DOCTYPE html>
<html>
<head>
<title>SDK Javascript Example</title>
<meta charset="UTF-8" />
</head>
<body>
<script
async
src="https://sdk.locallogic.co/sdks-js/<version>/index.umd.js"
onload="loadNeighborhoodSchoolsSDK()"
></script>
<style>
#neighborhood-schools-widget {
height: 700px;
width: 100%;
}
</style>
<!--NOTE: If you are implementing multiple SDKs, make sure you create unique IDs for each-->
<div id="neighborhood-schools-widget"></div>
<script>
const globalOptions = {
locale: "en", // Change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
function loadNeighborhoodSchoolsSDK() {
// Your API key or token
const ll = LLSDKsJS("your-api-key-here", globalOptions);
// This is the div that will contain the widget
const sdkContainer = document.getElementById("neighborhood-schools-widget");
const sdkOptions = {
geographyId: "g10_drt2yt86"
// ...Other sdk specific options
}
const sdkInstance = ll.create("neighborhood-schools", sdkContainer, sdkOptions);
}
</script>
</body>
</html>
Configurationβ
API Keyβ
Your API Key will be provided to you by the LocalLogic team.
Global optionsβ
const localLogicClient = localLogicSDK(apiKey, globalOptions)
These options are available accross SDKs. You will also be able to specify SDK specific options depending on the SDK you are implementing. These options will be available in the SDK specific documentation
Name | Required | Type | Default | Description |
---|---|---|---|---|
apiKey | true | string | ApiKey required for making requests to the Local Logic API. | |
globalOptions.appearance | false | Appearance | The appearance option provides theme and variable support customizing the look and feel of your widgets. | |
globalOptions.locale | false | "en" or "fr" | "en" | The locale option specifies the language of the scores and the UI interface. |
globalOptions.externalId | false | string | For SDKs connected to the Neighborhood Management App in the Local Logic Hub. This field is required for Neighborhood Showcase and Favorite Neighborhoods SDKs to work |
Appearanceβ
Local Logic SDKs support visual customization using the Appearance API, which allows you to match the look of the SDK to your brand.
type Appearance = {
theme?: "day" | "night"; // Defaults to "day"
variables?: {
[key: string]: string;
};
}
Commonly used variablesβ
Variable | Description |
---|---|
--ll-color-primary | The primary brand color. |
--ll-color-primary-variant1 | A slightly darker version of the primary brand colour. This variable should always be changed in conjunction with --ll-color-primary . |
--ll-font-family | Changes the font family used throughout the SDKs. Currently, the SDKs only support system fonts. This value should always include a fallback font family, ex. Inter, sans-serif . |
--ll-font-size-base | Used to scale the font size up or down. |
--ll-spacing-base-unit | Used to scale the overall padding of the SDKs. |
Functionsβ
Once you initialize your SDK client with your API key and global options, several functions are available.
Createβ
const sdkInstance = localLogicClient.create("neighborhood-schools", container, sdkOptions)
This function creates a new SDK widget based on the name specified.
sdkOptions
are specific to each SDK being created.
Name | Required | Type | Default | Description |
---|---|---|---|---|
sdkType | true | string | The SDK you would like to create. "neighborhood-schools" in this case. | |
container | true | HTMLElement | The element to render in to. | |
sdkOptions | true | SDKOptions | Options required for te specified sdkType . Options are detailed below. |
SDKOptionsβ
Name | Required | Type | Default | Description |
---|---|---|---|---|
sdkOptions.geographyId | * | string | The Local Logic geography ID. This is the preferred instantiation method. * This field is required if you are not using lat/lng . | |
sdkOptions.lat | * | number | Initial viewport latitude. * This field is required if you are not using geographyId . If geographyId is defined, this field is ignored. | |
sdkOptions.lng | * | number | Initial viewport longitude. * This field is required if you are not using geographyId . If geographyId is defined, this field is ignored. |
Updateβ
sdkInstance.update(sdkOptions)
This function is used to update the widget with new values. This can be useful when, for example, you want to change the widget location.
The sdkOptions
object follows the same structure as on creation.
Onβ
sdkInstance.on(event, callback)
This function takes a callback which is triggered when an event
occurs. Currently, there is only one event.
Name | Required | Type | Default | Description |
---|---|---|---|---|
event | true | "change" | The name of the event . | |
callback | true | CallbackFunction | The callback to be triggered when the specified event occurs. |
type CallbackFunction = ({ type: string, data: unknown }) => void;
Destroyβ
sdkInstance.destroy()
This function is used to teardown the created SDK widget.
Supporting API Endpointsβ
The following Local Logic APIs are used by the SDK to display data.
API | Description |
---|---|
Schools | Used to retrieve the schools within and close to the neighborhood. |