Local Content
Provide a comprehensive understanding of the location of any property in the US and Canada and an overview of the characteristics that matter most to the home buyer and renter.
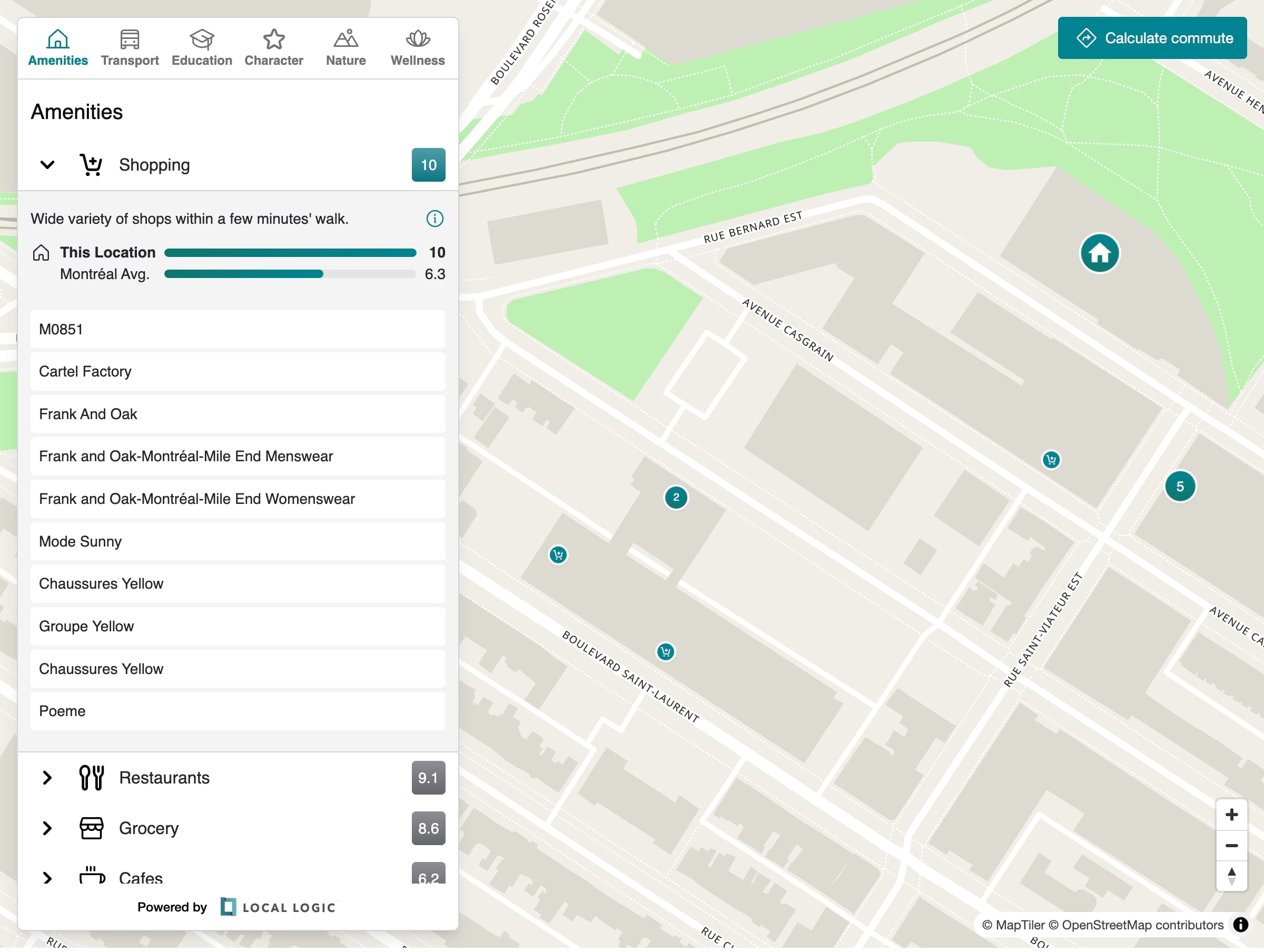

Installationβ
Our SDK products are served from a single source.
- React
- React Native
- Vanilla JavaScript
The SDKs are designed to work with React and other declarative frameworks.
First, install the entry script with npm
, yarn
, or pnpm
:
npm i --save @local-logic/sdks-js
yarn add @local-logic/sdks-js
pnpm add @local-logic/sdks-js
Example
import { useRef, useEffect } from "react";
import LLSDKsJS from "@local-logic/sdks-js";
const globalOptions = {
locale: "en", // If available, change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-border-radius-small": "8px",
"--ll-border-radius-medium": "16px",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
const localLogicClient = LLSDKsJS("your-api-key-here", globalOptions);
function LocalContentSDKComponent() {
const containerRef = useRef(null);
useEffect(() => {
if (!containerRef.current) {
return;
}
const sdkOptions = {
// Set the lat and lng of the location
lat: 45.5282164,
lng: -73.5978527,
// ...Other sdk specific options
}
const sdkInstance = localLogicClient.create(
"local-content",
containerRef.current,
sdkOptions,
);
// It is important to destroy the SDK instance when the component is unmounted.
return () => {
sdkInstance.destroy();
}
}, []);
return (
<div
ref={containerRef}
style={{
// Height is dependent on the SDK you are implementing
height: "700px",
width: "100%",
}}
/>
);
}
export default LocalContentSDKComponent;
The Local Logic React Native SDK component allows you to easily add one of our SDKs to your native Android and iOS apps using React Native.
First, install the entry script with npm
, yarn
, or pnpm
:
npm i --save @local-logic/sdks-js
yarn add @local-logic/sdks-js
pnpm add @local-logic/sdks-js
Install React Native Webview dependency
React Native Webview is used to port Local Logic SDKs to React Native.
Expo CLI
Install supported react-native-webview
version for Expo applications using Expo CLI.
npx expo install react-native-webview
React Native CLI
Install react-native-webview
using your project's package manager:
npm i --save react-native-webview
yarn add react-native-webview
pnpm add react-native-webview
If using CocoaPods, in the ios/
or macos/
directory run:
pod install
Usage
The React Native component accepts the same options as our web SDKs via it's options
, appearanceOptions
, and renderOptions
props.
import LLSDKs from "@local-logic/sdks-react-native";
const globalOptions = {
locale: "en", // Change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-border-radius-small": "8px",
"--ll-border-radius-medium": "16px",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
const sdkOptions = {
// Set the lat and lng of the location
lat: 45.5282164,
lng: -73.5978527,
// ...Other sdk specific options
};
<LLSDKs
apiKey="your-api-key-here"
sdkType="local-content"
options={sdkOptions}
appearanceOptions={globalOptions}
renderOptions={{ lazy: false }}
/>
The script is available in umd
and es
format from the Local Logic CDN: https://sdk.locallogic.co/sdks-js/<VERSION>/index.<FORMAT>.js
<VERSION>
is the latest script version number (which you can find on npm), and <FORMAT>
is either umd
or es
.
Example
<!DOCTYPE html>
<html>
<head>
<title>SDK Javascript Example</title>
<meta charset="UTF-8" />
</head>
<body>
<script
async
src="https://sdk.locallogic.co/sdks-js/<version>/index.umd.js"
onload="loadLocalContentSDK()"
></script>
<style>
#local-content-widget {
height: 500px;
width: 100%;
}
</style>
<div id="local-content-widget"></div>
<script>
const globalOptions = {
locale: "en", // Change to either english or french
appearance: {
theme: "day",
// Add any other appearance changes here
variables: {
"--ll-color-primary": "#fd3958",
"--ll-color-primary-variant1": "#d5405b",
"--ll-border-radius-small": "8px",
"--ll-border-radius-medium": "16px",
"--ll-font-family": "Avenir, sans-serif"
}
}
};
function loadLocalContentSDK() {
(async () => {
// Your API key or token
const ll = LLSDKsJS("your-api-key-here", globalOptions);
const container = document.createElement("div");
// NOTE: If you are implementing multiple SDKs, make
// sure you create unique IDs for each
container.setAttribute("id", "local-content-widget");
// Set the styles of the container
container.style.cssText = `
// Height is dependent on the SDK you are implementing
height: 700px;
width: 100%;
display: flex;
`;
// This is the div that will contain the widget
document.querySelector("#local-content-widget").appendChild(container);
const sdkOptions = {
// Set the lat and lng of the location
lat: 45.528126,
lng: -73.598104,
// ...Other sdk specific options
}
const sdkInstance = ll.create("local-content", container, sdkOptions);
})();
}
</script>
</body>
</html>
Configurationβ
API Keyβ
Your API Key will be provided to you by the LocalLogic team.
If you are migrating from an old Local Logic SDK product, the API keys from the legacy versions do not work with our existing products.
Please reach out to Local Logic support to get your new key.
Global optionsβ
const localLogicClient = localLogicSDK(apiKey, globalOptions)
These options are available accross SDKs. You will also be able to specify SDK specific options depending on the SDK you are implementing. These options will be available in the SDK specific documentation
Name | Required | Type | Default | Description |
---|---|---|---|---|
apiKey | true | string | ApiKey required for making requests to the Local Logic API. | |
globalOptions.appearance | false | Appearance | The appearance option provides theme and variable support customizing the look and feel of your widgets. | |
globalOptions.locale | false | "en" or "fr" | "en" | The locale option specifies the language of the scores and the UI interface. |
Appearanceβ
Local Logic SDKs support visual customization using the Appearance API, which allows you to match the look of the SDK to your brand.
type Appearance = {
theme?: "day" | "night"; // Defaults to "day"
variables?: {
[key: string]: string;
};
}
Commonly used variablesβ
Variable | Description |
---|---|
--ll-color-primary | The primary brand color. |
--ll-color-primary-variant1 | A slightly darker version of the primary brand colour. This variable should always be changed in conjunction with --ll-color-primary . |
--ll-font-family | Changes the font family used throughout the SDKs. Currently, the SDKs only support system fonts. This value should always include a fallback font family, ex. Inter, sans-serif . |
--ll-font-size-base | Used to scale the font size up or down. |
--ll-spacing-base-unit | Used to scale the overall padding of the SDKs. |
Functionsβ
Once you initialize your SDK client with your API key and global options, several functions are available.
Createβ
const sdkInstance = localLogicClient.create("local-content", container, sdkOptions)
This function creates a new SDK widget based on the name specified.
sdkOptions
are specific to each SDK being created.
Name | Required | Type | Default | Description |
---|---|---|---|---|
sdkType | true | string | The SDK you would like to create. "local-content" in this case. | |
container | true | HTMLElement | The element to render in to. | |
sdkOptions | true | SDKOptions | Options required for te specified sdkType . Options are detailed below. |
SDKOptionsβ
Name | Required | Type | Default | Description |
---|---|---|---|---|
sdkOptions.lat | true | number | Initial viewport latitude. | |
sdkOptions.lng | true | number | Initial viewport longitude. | |
sdkOptions.marker | true | Marker | Will render a pin marker on the map representing the lat/lng. The marker can be used to symbolize a location being analyzed. | |
sdkOptions.zoom | false | number | 16 | Initial viewport zoom. |
sdkOptions.pitch | false | number | 0 | Initial viewport pitch. |
sdkOptions.bearing | false | number | 0 | Initial viewport bearing. |
sdkOptions.cooperativeGestures | false | boolean | true | If true, scroll zoom will require pressing the ctrl or β key while scrolling to zoom map, and touch pan will require using two fingers while panning to move the map. |
sdkOptions.distanceUnit | false | DistanceUnit | metric | Primarily used for the commute calculator. Will display the distance in mi/yd under the imperial system or km/m under the metric system. |
sdkOptions.mapProvider | false | MapProvider | { name: "maptiler" } | Desired map provider data; key is only required if name is set to "google". |
sdkOptions.hideScores | false | boolean | false | Specifies whether or not scores should be hidden. NOTE: If set to true, scores without POIs won't be displayed. |
sdkOptions.collapseScores | false | boolean | false | Specifies whether or not the first score inside a category should be auto-expanded on load. If set to false, all score accordions will be collapsed by default. |
Updateβ
sdkInstance.update(sdkOptions)
This function is used to update the widget with new values. This can be useful when, for example, you want to change the widget location.
The sdkOptions
object follows the same structure as on creation.
Onβ
sdkInstance.on(event, callback)
This function takes a callback which is triggered when an event
occurs. Currently, there is only one event.
Name | Required | Type | Default | Description |
---|---|---|---|---|
event | true | "change" | The name of the event . | |
callback | true | CallbackFunction | The callback to be triggered when the specified event occurs. |
type CallbackFunction = ({ type: string, data: unknown }) => void;
Destroyβ
sdkInstance.destroy()
This function is used to teardown the created SDK widget.
Mapsβ
The SDK currently supports 2 maps: MapTiler and Google Maps.
- MapTiler
- Google Maps
MapTiler is used by default and the map api key belongs to Local Logic.
If you wish to use Google Maps, you will need to supply your google api key when implementing the SDK.
Extra steps for enabling Google Maps supportβ
-
Retrieve your Google Maps API key from your Google Cloud Platform project. If you do not have an API key, here are instructions on how to set one up.
-
Within your Google Cloud Platform project, please have the following APIs enabled for use:
- Maps JavaScript API
- Routes API
- Places API
For instructions on how to enable Places API for example, see this link.
-
Add the
mapProvider
param within your SDK container configuration. The value for thename
key should be set to"google"
. Your Google Maps API key should be provided underkey
. Please see the following as an example configuration:
Example
const sdkOptions = {
// Set the lat and lng of the location
lat: 45.5282164,
lng: -73.5978527,
// ...Other sdk specific options
mapProvider: {
name: "google",
key: "your-api-key-here",
},
};
localLogicSDK.create("local-content", container, sdkOptions);
Type definitionsβ
Marker typeβ
// Typically the same as lat / lng provided in the main options
type Marker = {
lat: number;
lng: number;
};
DistanceUnit typeβ
type DistanceUnit = "metric" | "imperial";
MapProvider typeβ
type MapProvider = {
name: "maptiler" | "google",
key?: string; // Required when "google"
};